R Markdown Best Practices
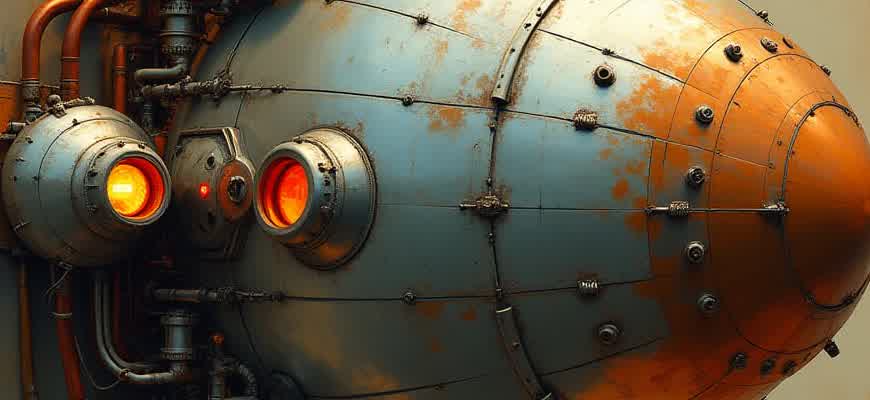
R Markdown is a powerful tool for combining code, results, and narrative in a single document. To maximize its effectiveness, following structured practices is essential. Below are some key guidelines for creating clean, reproducible, and well-organized R Markdown files:
- Organize code chunks efficiently: Group related code into separate chunks for readability and maintenance. Avoid long, complex code blocks that are difficult to debug.
- Use meaningful chunk labels: Label your chunks descriptively to provide context for each section of the code.
- Ensure reproducibility: Provide all necessary data and functions within the R Markdown file to ensure it can be run on any machine without external dependencies.
Key Best Practices:
Practice | Description |
---|---|
Clear Documentation | Document each step of the analysis to enhance clarity and reproducibility. Use markdown cells to explain the logic behind the code. |
Consistent Formatting | Maintain consistent formatting throughout the document to improve readability and flow of information. |
Remember, a well-documented R Markdown file not only communicates your analysis effectively but also makes it easier for others to replicate and understand your work.
How to Structure Your R Markdown File for Future Expansion
Creating R Markdown documents that can scale efficiently is essential for managing larger projects and teams. A well-organized document not only facilitates easier collaboration but also ensures that your analysis remains reproducible and maintainable over time. To achieve this, it's crucial to keep in mind both the layout and modularity of your code, as well as the clarity of your outputs. The following recommendations will help you structure your R Markdown file for greater flexibility and growth.
One of the key practices in organizing your document is separating different components into logical sections. This can include data preparation, analysis, and result visualization. Structuring the content using headers and clear blocks of code ensures that your document remains understandable and manageable as the project evolves.
1. Use Sectioning and Clear Code Blocks
- Organize your R Markdown into distinct sections with meaningful headers (e.g., "Data Preprocessing", "Modeling", "Results").
- Each section should start with a heading and be followed by a well-documented code chunk. This allows others to navigate easily through your document.
- Consider breaking complex code into smaller, reusable chunks to improve readability and maintainability.
2. Modularize the Code with External Scripts
- For lengthy code, it's a good practice to link to external R scripts instead of placing everything directly into the R Markdown file. This keeps your document cleaner and makes it easier to maintain.
- Use the
source()
function to call external scripts when necessary. This promotes code reusability and simplifies debugging. - External scripts should be organized logically, such as by function (e.g., "data_cleaning.R", "model_training.R").
3. Include Key Information for Readability and Scalability
Best Practice | Benefit |
---|---|
Document functions and code chunks | Improves collaboration and future editing. |
Use inline comments for clarification | Increases code understandability for others and your future self. |
“A clear structure not only aids in scalability but also makes your R Markdown document more collaborative, allowing others to contribute with ease.”
Optimizing Code Chunks for Better Performance and Readability
When working with R Markdown documents, efficient code execution and clear, readable output are critical for maintaining quality and ensuring that analyses are both reproducible and understandable. Code chunks in R Markdown serve as a central component for integrating code and results within the document, but improperly optimized chunks can slow down performance and make your work harder to follow. It is essential to manage the execution environment and formatting strategies effectively for improved usability and speed.
To enhance both performance and readability, it’s important to adopt strategies that streamline code execution and keep the document clean and organized. This includes controlling output verbosity, ensuring that chunks are as efficient as possible, and using appropriate chunk options to optimize results presentation. The following best practices focus on these aspects.
Best Practices for Code Chunk Optimization
- Minimize unnecessary output: Use the
echo = FALSE
option for code that doesn't need to be shown in the final document but is essential for calculations. - Use chunk caching: Caching results with
cache = TRUE
is a great way to speed up document rendering by skipping the re-execution of unchanged chunks. - Keep chunks focused: Break larger chunks into smaller, more manageable pieces. This makes it easier to troubleshoot and improves code readability.
- Control error handling: Prevent code from halting the document rendering process with
error = TRUE
orwarning = TRUE
when testing code in non-critical sections.
Strategies for Readable and Maintainable Code
- Comment and document effectively: Always add comments to your code to explain the logic and any assumptions. This is vital for others (or yourself) when revisiting the analysis after some time.
- Use meaningful variable names: Avoid single-letter variables like
x
ory
. Instead, use descriptive names that indicate the data they represent. - Consistent code style: Follow a coding style guide (e.g.,
styler
orlintr
) to ensure uniformity across your code chunks.
Tip: It’s recommended to usemessage = FALSE
andwarning = FALSE
for code chunks that do not provide valuable information, to keep the document clean.
Chunk Execution Order and Performance
Strategy | Description |
---|---|
Parallel Execution | Enable parallel processing using the future package to execute code chunks simultaneously, reducing overall processing time. |
Chunk Grouping | Group related computations together. This avoids redundant recalculations and speeds up document rendering by maintaining efficient chunk dependencies. |
Approaches to Managing Large Datasets in R Markdown
Working with large datasets in R Markdown requires efficient strategies to ensure smooth document rendering and optimal performance. Given the limitations of system resources and the complexity of large datasets, it’s essential to adopt methods that allow for effective analysis without overwhelming memory or causing excessive processing time. Below are key strategies to handle large datasets efficiently in R Markdown reports.
One effective approach is to utilize data preprocessing techniques to reduce the size of the dataset before loading it into the R Markdown document. Additionally, leveraging data storage formats that are optimized for performance, such as databases or specialized file types, can help manage data more efficiently. Combining these techniques ensures that large datasets can be worked with in a manageable and reproducible way.
1. Use of Data Sampling and Chunking
- Consider using a random sample of the data for initial analysis or visualizations. This allows for faster processing without compromising the insights drawn from a large dataset.
- Split data into smaller chunks to process and analyze in parts. This reduces memory consumption and speeds up calculations.
- For larger operations, consider loading and processing data in separate chunks or through external scripts that interact with the main R Markdown document.
2. Efficient Data Formats
When working with large datasets, it is crucial to choose the right data format for storage and processing. Formats like CSV can be slow to read and write for large datasets, whereas binary formats like RDS or database-backed storage (e.g., SQLite) offer faster I/O operations.
Tip: Using R's data.table package can significantly speed up data manipulation tasks by enabling efficient memory use and fast data processing.
3. Parallel Processing and Memory Management
For time-intensive computations, consider parallel processing by using packages such as future or parallel. These packages enable the use of multiple cores or machines, speeding up large computations.
- Use parallel::mclapply() for parallel processing of data transformations.
- Make use of future.apply to apply functions over large datasets using parallel computation.
4. Storing Intermediate Results in Cache
When working with multiple analyses on large datasets, storing intermediate results in a cache file can save time and prevent redundant computations. This strategy can be implemented using the cache chunk option in R Markdown.
Strategy | Benefit |
---|---|
Data Sampling | Reduces processing time without sacrificing analysis quality. |
Efficient Data Formats | Optimizes reading and writing of large datasets. |
Parallel Processing | Speeds up computational tasks by utilizing multiple cores. |
Intermediate Result Caching | Prevents redundant computations and speeds up report rendering. |
Adapting Output Formats in R Markdown for Different Audiences
When working with R Markdown, tailoring the output format is essential for ensuring your analysis meets the needs of your audience. Whether you're preparing a report for a non-technical stakeholder or a detailed document for an academic audience, adjusting the structure and presentation of the content can greatly enhance its clarity and impact. R Markdown allows a wide range of customization options, enabling you to choose formats and elements that are most effective for different readers.
One of the key strategies in customizing output formats is modifying the structure, style, and type of content presented. This can include adjusting the level of detail, choosing appropriate visualizations, and selecting the best layout for specific readers. For instance, non-technical audiences might prefer a high-level summary with key figures, while technical audiences may require in-depth tables and code snippets. Below are some techniques to consider for optimizing your R Markdown outputs.
Key Customization Techniques
- Text-Level Customization: Simplify or enrich the language used in the text, depending on the target audience. For non-technical users, limit jargon and use more narrative-style explanations.
- Visual Adjustments: Choose visuals that best communicate the data. For example, graphs with minimal text are often sufficient for broader audiences, while more complex plots with annotations are appropriate for analytical readers.
- Conditional Output: Use the `knitr` chunk options to selectively display content based on output format or audience. For example, you can hide technical details or include them only for certain formats like HTML or PDF.
Formatting for Specific Audiences
- Non-Technical Stakeholders:
- Focus on summaries and conclusions.
- Highlight key findings in bulleted lists and simple tables.
- Use clear, simple charts (e.g., bar plots, pie charts) to illustrate trends.
- Technical Audience:
- Incorporate detailed tables with raw data.
- Use more sophisticated plots (e.g., scatter plots, heatmaps) with annotations and legends.
- Provide access to the underlying R code for reproducibility.
Note: Be mindful that customizing the output format requires balancing between simplicity for non-technical users and the depth of analysis expected by a technical audience. The right format ensures clarity without overwhelming the reader with unnecessary details.
Example Table: Tailoring Layouts
Audience | Recommended Output | Visual Elements |
---|---|---|
Non-Technical Stakeholders | High-level summaries, key insights | Simple bar charts, bullet-point lists |
Technical Audience | Detailed analysis, code snippets | Complex plots, tables with raw data |
Enhancing Reports with Interactivity in R Markdown
R Markdown is not only a powerful tool for dynamic reporting, but it also supports the integration of interactive elements that can significantly enrich the user's experience. By using interactive components, analysts can create more engaging, user-driven reports. These features allow users to explore the data themselves, offering a deeper understanding of the findings and trends presented.
Incorporating interactive elements into R Markdown reports can be achieved through a variety of tools and packages. One popular option is the `plotly` package, which transforms static visualizations into interactive plots. Additionally, the `leaflet` package allows users to create interactive maps, while `DT` makes tables dynamic with features like sorting and filtering. These interactive elements can be embedded directly within the R Markdown document, offering users a rich, hands-on experience.
Key Interactive Features to Use in R Markdown
- Interactive Plots - With packages like `plotly`, users can hover over, zoom in, and explore the data points in plots.
- Dynamic Tables - Using the `DT` package, you can create sortable and searchable tables that adjust to user input.
- Maps - The `leaflet` package allows you to generate maps that users can zoom into, click on, and get more detailed geographic information.
Best Practices for Interactive Content
- Keep it Simple - While interactive elements can be exciting, too many can overwhelm users. Focus on key features that add value.
- Ensure Compatibility - Make sure the interactive elements are compatible with the format you're rendering (e.g., HTML, PDF, Word) and function properly across different platforms.
- Test Interactivity - Before finalizing the report, test all interactive components to ensure they work as expected and provide a smooth user experience.
Tip: Interactivity should be used to complement the narrative, not distract from it. Use it to highlight key insights, not as a decorative feature.
Example of Interactive Table
Country | Population | GDP |
---|---|---|
USA | 331 Million | $21 Trillion |
China | 1.4 Billion | $14 Trillion |
Germany | 83 Million | $4 Trillion |
Managing Dependencies and External Resources in R Markdown Projects
Efficient handling of dependencies and external resources is crucial for ensuring the reproducibility and smooth execution of R Markdown documents. By properly managing these elements, you can avoid issues such as missing libraries or outdated files, which can lead to inconsistent results. This process involves organizing both the R package dependencies and external resources like images, datasets, or scripts used within the R Markdown project.
One of the most effective ways to manage dependencies is through the use of a renv or packrat package, which ensures that all required libraries are locked to specific versions for the project. By doing so, you create a controlled environment that guarantees that every time the document is knitted, the results remain consistent across different systems and setups.
Using `renv` for Dependency Management
To begin, you can initialize a new environment in your R project directory by running the following command in the R console:
renv::init()
This creates an renv.lock file that records the versions of all the packages in use. When others open your R Markdown project, they can use the renv::restore()
function to automatically install the exact versions of the packages you used.
Managing External Resources
External resources, such as datasets, images, or custom scripts, should be organized within the project directory to ensure accessibility and reproducibility. These resources can be included in the R Markdown document using relative file paths, which helps avoid issues related to absolute paths that might change depending on the machine. Here are some tips:
- Keep resources like images or CSV files in a subfolder (e.g., assets) within the project directory.
- Reference files using relative paths to avoid broken links when sharing the document.
- Use functions like
knitr::include_graphics()
to embed images directly into the document.
Key Considerations for External Files
When working with large external datasets or files, it is important to consider their impact on the document’s size and performance. You can compress data files or use remote sources to reduce the load.
Always ensure that any external file is included in version control, or provide clear instructions for others on how to access the resources if they are hosted externally.
Example Setup
A typical project structure might look like this:
Folder/File | Description |
---|---|
project.Rmd | R Markdown file containing the main analysis and report. |
assets/ | Folder containing images and datasets used in the project. |
renv.lock | File that tracks package dependencies for reproducibility. |
Debugging and Troubleshooting Common Issues in R Markdown
When working with R Markdown, errors can often arise, disrupting the flow of your work. Identifying and fixing these issues is crucial for producing accurate reports. Many problems in R Markdown are related to R code execution or incorrect syntax in markdown formatting. Understanding the root causes of these issues and knowing how to resolve them can save time and enhance the overall experience.
Common problems in R Markdown include issues with rendering chunks, incorrect chunk options, or conflicts between code and markdown elements. Debugging these errors efficiently requires a systematic approach to identify where the problem originates. Below are some key troubleshooting steps and tips to resolve typical issues that users encounter in R Markdown documents.
Key Debugging Steps
- Check for Errors in R Code: Make sure that your R code runs correctly in a standalone R script before embedding it in a markdown chunk. Use the knitr package to isolate the issue.
- Review Chunk Options: Incorrect chunk options such as eval or echo may prevent code from running as expected. Ensure that options like message = FALSE or warning = FALSE are appropriately used to suppress unnecessary output.
- Verify Markdown Syntax: Ensure that markdown syntax for headers, lists, and tables is correctly formatted. Improper formatting can cause rendering issues.
Common Errors and Their Solutions
- Error: 'knit' function not found: This occurs when the knitr package is not loaded. Solution: Ensure the knitr package is installed and loaded using
library(knitr)
. - Error: Code chunk not rendering correctly: This may be due to incorrect chunk delimiters or missing chunk options. Solution: Double-check that each chunk starts with
```{r}
and ends with```
. - Error: Invalid chunk options: This can happen when you use an unrecognized option in a code chunk. Solution: Review the documentation for available chunk options and ensure they are spelled correctly.
Table of Common Issues and Solutions
Problem | Solution |
---|---|
Missing packages | Use install.packages() to install required packages. |
Inconsistent output from R code | Check for any discrepancies between interactive R console results and R Markdown execution. |
Render errors in HTML output | Ensure proper HTML tags and escape sequences are used. |
Remember that debugging in R Markdown often involves ensuring that both R code and markdown formatting are correct. Identifying which part of your document is causing the issue–whether it's the code execution or markdown formatting–is essential to a quick resolution.